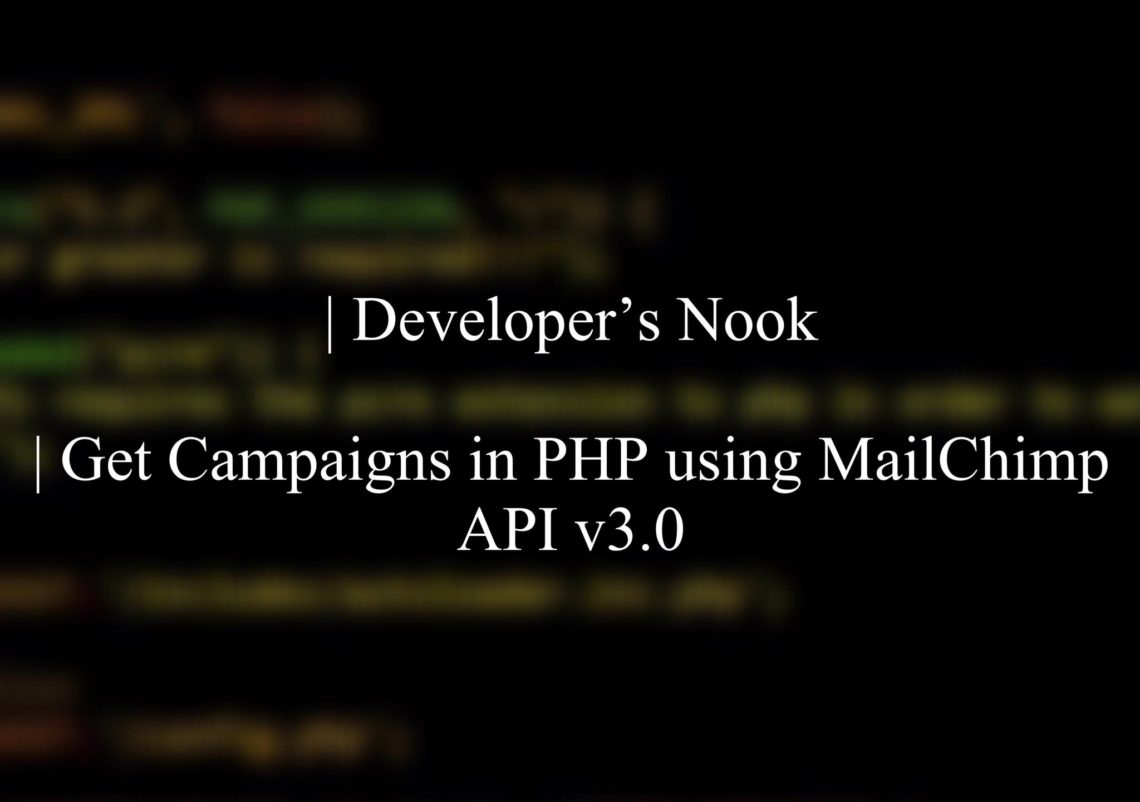
How to Get Campaigns in MailChimp API v3.0 using PHP cURL
In this blog, we will discuss and show you how you can get campaigns in MailChimp API v3.0 using PHP cURL.
function mailchimp_curl_connect( $url, $request_type, $api_key, $data = array() ) {
if( $request_type == 'GET' )
$url .= '?' . http_build_query($data);
$mch = curl_init();
$headers = array(
'Content-Type: application/json',
'Authorization: Basic '.base64_encode( 'user:'. $api_key )
);
curl_setopt($mch, CURLOPT_URL, $url );
curl_setopt($mch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($mch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($mch, CURLOPT_CUSTOMREQUEST, $request_type);
curl_setopt($mch, CURLOPT_TIMEOUT, 10);
curl_setopt($mch, CURLOPT_SSL_VERIFYPEER, false);
if( $request_type != 'GET' ) {
curl_setopt($mch, CURLOPT_POST, true);
curl_setopt($mch, CURLOPT_POSTFIELDS, json_encode($data) );
return curl_exec($mch);
}
We will use the function mailchimp_curl_connect
to connect to Mailchimp API v3.0 via cURL request, with the following parameters:
- $url: The API endpoint
- $request_type: GET, POST, PATCH and DELETE
- $api_key: API key from Mailchimp
- $data: API parameters
Example request:
$data = array(
'count' => 50, // the number of lists to return, default - all
'before_create_time' => '2014-02-01', // only lists created before this date
'after_date_created' => '2014-02-05' // only lists created after this date
'list_id' => '123456', //list id
'status' => 'save',
'sort_field' => 'create_time',
'sort_dir' => 'ASC'
);
The parameters on the array $data are filter fields from the Campaign API. https://mailchimp.com/developer/reference/campaigns/
$api_key = 'YOUR API KEY HERE'; $url = https://{API PREFIX}.api.mailchimp.com/3.0/campaigns/
API prefix is the last part of your API key, for exampleus5
,us8
etc. https://mailchimp.com/help/about-api-keys/
$result = json_decode( mailchimp_curl_connect( $url, 'GET', $api_key, $data) );
We can print the result by:
echo "<pre>";
print_r($result);
This post on how to get campaigns in PHP using MailChimp API v3.0 will be the first of many guides that we will publish under “Developer’s Nook” to help fellow programmers who will also encounter the same concerns as mine. We will try to document and share all the problems and resolutions we encounter at work as much as we can.

